728x90
redux의 구성
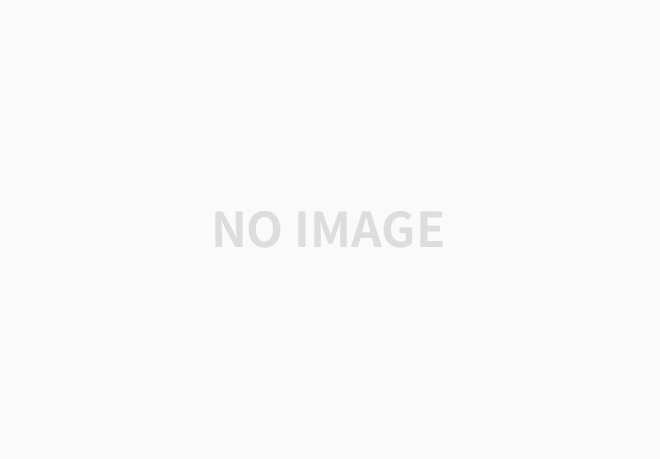
- Action : string
- action type 지정
- Action Creator : function(return object)
- Action Type과 payload 를 반환
- Reducer : function(return state mutation)
- state(initialstate) 와 action은 parameter로 받음
- Store
- createStore 로 생성
- reducer 와 middleware를 매개 변수로 받음
- Middleware
- action과 reducer 사이에 중간 처리해주는 함수
- logger, thunk등
다음은 위의 redux 기본구조를 구현한 모습니다. (middleware는 생략)
const { createStore, combineReducers } = require('redux');
const produce = require('immer').produce;
//action && action creator
const ADD_CAKE = 'ADD_CAKE';
const orderCake = () => ({
type: ADD_CAKE,
quantity: 1,
});
const ADD_ICECREAM = 'ADD_ICECREAM';
const orderIceCream = (q) => ({
type: ADD_ICECREAM,
quantity: q,
});
//initialState
const cakeInitialState = {
//immer 시현용으로 일부러 nested
nested: {
numOfCakes: 10,
},
};
const icecreamInitialState = {
numOfIcecreams: 10,
};
//reducer
const cakeReducer = (state = cakeInitialState, action) => {
switch (action.type) {
case 'ADD_CAKE':
//굳이 produce 쓸필요 없지만 nested object case 라 가정
return produce(state, (draft) => {
draft.nested.numOfCakes -= action.quantity;
});
default:
return state;
}
};
const icecreamReducer = (state = icecreamInitialState, action) => {
switch (action.type) {
case 'ADD_ICECREAM':
return {
...state,
numOfIcecreams: state.numOfIcecreams - action.quantity,
};
default:
return state;
}
};
//store && logging
const store = createStore(combineReducers({ cake: cakeReducer, icecream: icecreamReducer }));
console.log('initial state', store.getState());
const unsubscribe = store.subscribe(() => {
console.log(store.getState());
});
store.dispatch(orderCake());
store.dispatch(orderIceCream(2));
unsubscribe();
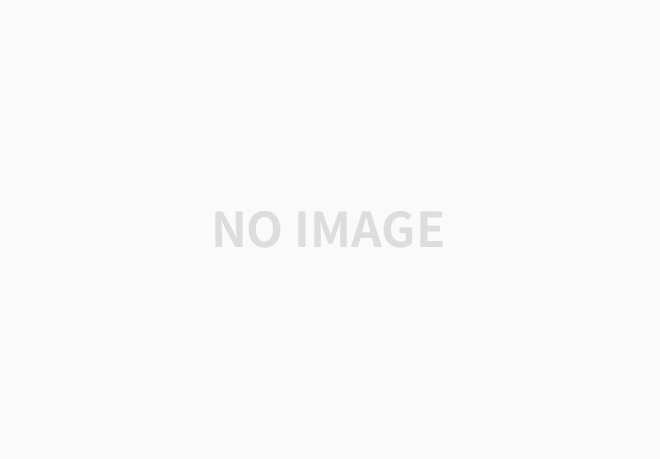
'React' 카테고리의 다른 글
Redux toolkit - extraReducers(2) - redux thunk (0) | 2022.05.15 |
---|---|
Redux toolkit - extraReducers(1) - module (0) | 2022.05.15 |
Redux toolkit - Redux toolkit 기본 (0) | 2022.05.15 |
blog 만들기 - husky v7, lint-staged, eslint, prettier (0) | 2022.04.12 |
React 18 변경 사항 (0) | 2022.04.11 |